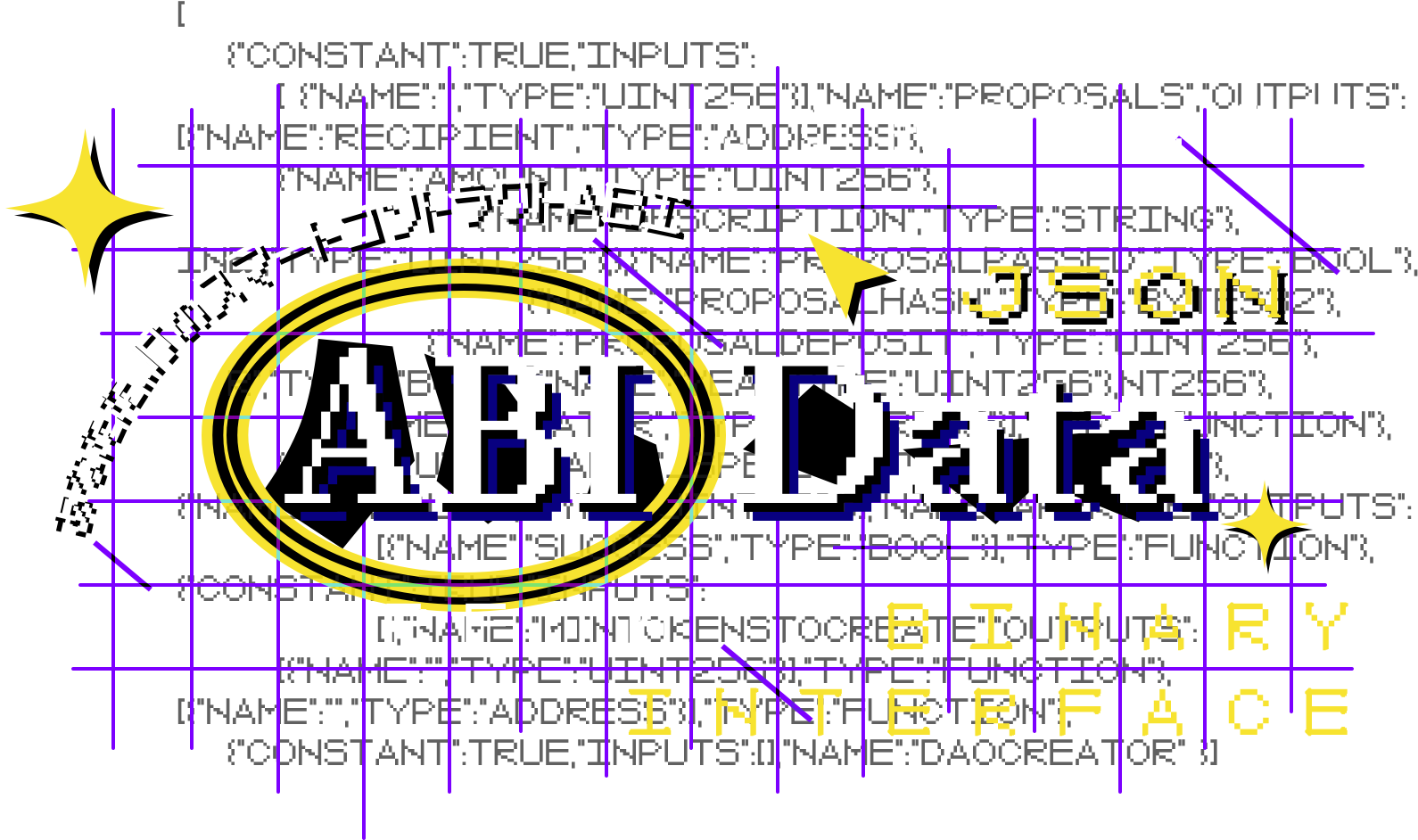
Remotely fetch smart contract ABI as JSON to use with wagmi and ethers.js in your app.
How do I use this API?
Append the contract address after abidata.net and optionally pass a network param, thatβs it. You donβt need any API keys.
Which networks are supported?
Name | Network ID |
---|---|
Ethereum Mainnet | none (default) |
Ethereum Goerli Testnet | goerli |
Ethereum Sepolia Testnet | sepolia |
Avalanche Mainnet | avalanche |
Avalanche Fuji Testnet | avalancheFuji |
Arbitrum Mainnet | arbitrum |
Arbitrum Goerli Testnet | arbitrumGoerli |
Arbitrum Nova | arbitrumNova |
Base Mainnet | base |
Base Goerli Testnet | baseGoerli |
BSC Mainnet | bsc |
BSC Testnet | bscTestnet |
Fantom Mainnet | fantom |
Fantom Testnet | fantomTestnet |
Polygon Mainnet | polygon |
Polygon Mumbai Testnet | polygonMumbai |
Polygon zkEVM Mainnet | polygonZkEvm |
Polygon zkEVM Testnet | polygonZkEvmTestnet |
Optimism Mainnet | optimism |
Optimism Goerli Testnet | optimismGoerli |
Gnosis Mainnet | gnosis |
Example:
https://abidata.net/<contract_address>?network=<network_id>
abidata.net/0xBB9bc244D798123fDe783fCc1C72d3Bb8C189413
abidata.net/0x9a879320A9F7ad2BBb02063d67baF5551D6BD8B0?network=goerli
abidata.net/0x779877a7b0d9e8603169ddbd7836e478b4624789?network=sepolia
React usage Example:
import { useState, useEffect } from 'react';
import { useContractWrite, usePrepareContractWrite } from 'wagmi';
const contractAddress = "0xecb504d39723b0be0e3a9aa33d646642d1051ee1";
const useContractABI = (contractAddress) => {
const [contractABI, setContractABI] = useState(null);
useEffect(() => {
const fetchContractABI = async () => {
const response = await fetch(`https://abidata.net/${contractAddress}`);
const json = await response.json();
setContractABI(json.abi);
};
fetchContractABI();
}, [contractAddress]);
return contractABI;
};
function App() {
const contractABI = useContractABI(contractAddress);
const { config } = usePrepareContractWrite({
address: contractAddress,
abi: contractABI,
functionName: 'feed',
});
const { data, isLoading, isSuccess, write } = useContractWrite(config);
return (
<div>
<button disabled={!write} onClick={() => write?.()}>
Feed
</button>
{isLoading && <div>Check Wallet</div>}
{isSuccess && <div>Transaction: {JSON.stringify(data)}</div>}
</div>
)
}
Is this free to use?
Yes, itβs free for everyone.
If you wanna support this project send some eth to ens.pug.eth
Can this be used in production?
Yes. Records are cached for 30 days, so after the initial request for a contract, the API will respond very quickly from the CDN.
Is the source code available?
Yes, check it out on GitHub. The main branch is automatically deployed to Vercel.